PowerShell Parameter Validation: Ensuring Valid Input for FunctionsPowerShell Parameter Validation: Ensuring Valid Input for Functions
Validating function parameters before executing any actions is a good idea. Learn these two techniques for performing parameter validation.
February 20, 2024
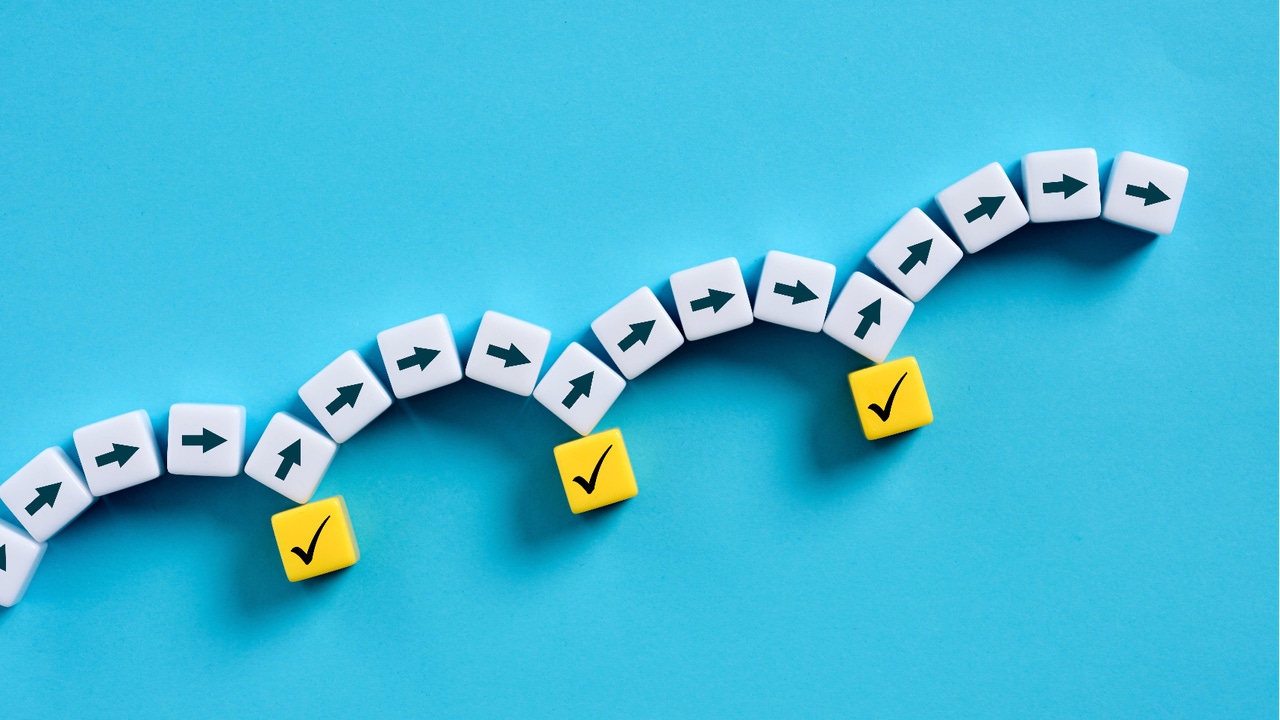
PowerShell makes it easy to pass values to functions using parameters. However, it is best practice to validate parameters before taking action to prevent unexpected values from causing unpredictable behavior.
That being the case, I want to share two methods I often use to validate the parameters passed to a function.
Before I begin, I want to point out that the techniques I’m about to demonstrate are only suitable for scenarios where you pass a string value to a function and are sure that the string should contain one of several possible values. If you are working with other types of data or don’t know ahead of time which values for the string are acceptable, you must resort to using other validation techniques.
#1. Validation Check Using the -Contains Operator
So, with that said, here is a very simple PowerShell script that demonstrates the first validation technique:
Function Display-ColorText {
param(
[String]$Color
)
$Colors = "Red","Green","Blue","Yellow","Gray"
if($Colors -contains $Color) {
Write-Host "This text is" $Color -ForegroundColor $Color
} else {
Write-Host $Color 'is not a Supported Color.'
}
}
$Color = Read-Host "Please type the name of a color"
Display-ColorText $Color
The script prompts the user to enter the name of a color. It then sends the entered color to a function called Display-ColorText. Of course, the user could enter just about anything, not necessarily a valid color name, including misspellings and unsupported color names. The script addresses this using a validation step to check if the entered color name is appropriate.
Looking at the script, you’ll notice that I created a variable called $Colors and set it to be equal to:
“Red”, “Green”, “Blue”, “Yellow”, “Gray”
These are the colors that the script will support. While PowerShell recognizes a broader range of color names, the script deliberately focuses on these five colors to keep things simple.
The next line of code checks if the color name entered by the user (stored in the $Color variable) exists in the list of supported colors defined by the $Colors variable. This check is based on the -Contains operator. If the color entered by the user is present in the list, the script outputs a line of text in the chosen color. Conversely, if the color is not supported, the user receives a message that says they selected an unsupported color. You can see an example of this for "Purple" in Figure 1.
Validate 1
Figure 1. This is what my script does.
#2. Validation Check Using ValidateSet
Now that I have demonstrated how to perform parameter validation using a -Contains operator, I will introduce a different technique that accomplishes more or less the same thing.
Here is the script:
Function Display-ColorText {
param(
[ValidateSet("Red","Green","Blue","Yellow","Gray”)]
[String]$Color
)
Write-Host "This text is" $Color -ForegroundColor $Color
}
$Color = Read-Host "Please type the name of a color"
Display-ColorText $Color
In this script, ValidateSet is used instead of the -Contains operator. Notice that all acceptable input values are defined within the Param section, meaning you don’t have to worry about writing a block of code to validate the input. The ValidateSet statement handles the validation process for you.
This technique works exceptionally well if the user enters a valid color. However, the script spews a lengthy and convoluted error message when the user input is invalid. Figure 2 shows an example of the error message.
Validate 2
Figure 2. Using ValidateSet works well until the user enters something invalid.
There is a way to handle errors gracefully, but error handling requires PowerShell 7 or higher.
Look at the script below. It’s identical to the previous script but adds an error message to the validation set. The cool thing about this error message is that it tells the user what values are acceptable input.
Function Display-ColorText {
param(
[ValidateSet("Red","Green","Blue","Yellow","Gray",ErrorMessage="The color {0} is invalid. Try using {1}")]
[String]$Color
)
Write-Host "This text is" $Color -ForegroundColor $Color
}
$Color = Read-Host "Please type the name of a color"
Display-ColorText $Color
Validate 3
Figure 3. PowerShell 7 greatly improves error handling.
About the Author
You May Also Like