How To Remove an Item From a PowerShell ArrayHow To Remove an Item From a PowerShell Array
Learn two methods – an easy way and a hard way – for removing items from PowerShell arrays.
December 1, 2023
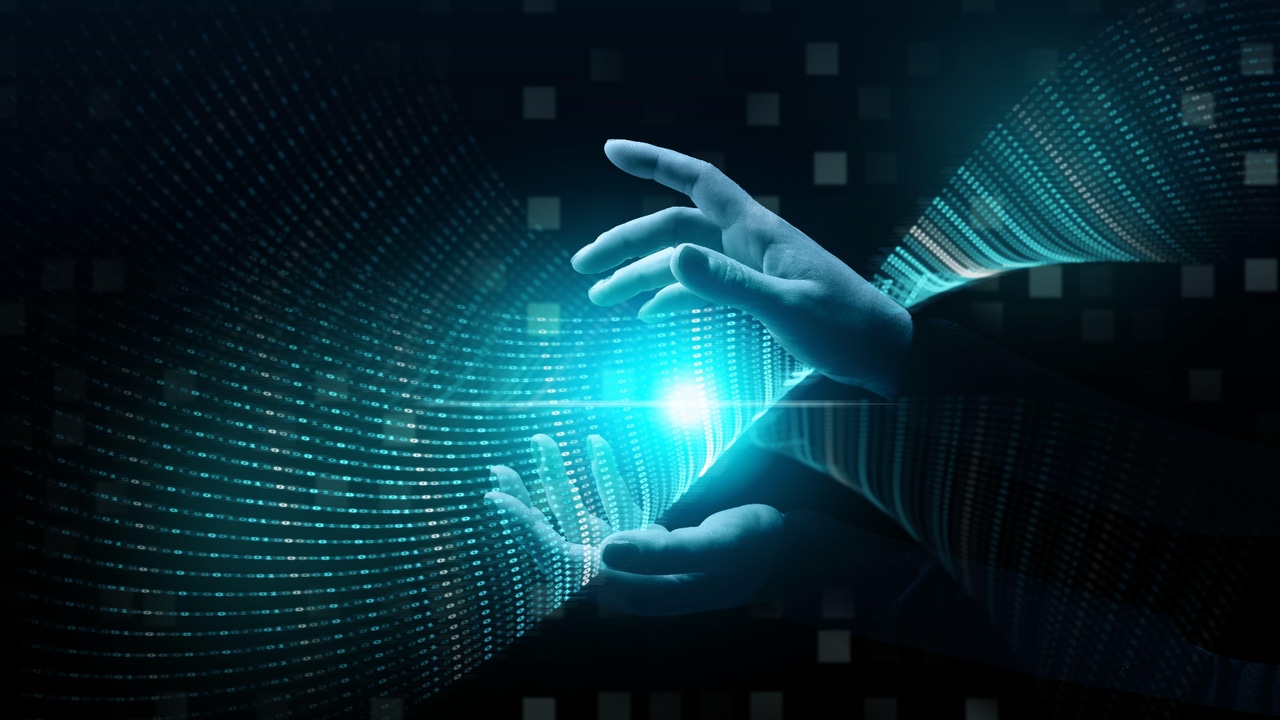
While most tasks involving arrays in PowerShell are relatively simple, removing an item from an array presents a challenge. PowerShell lacks a native cmdlet specifically for removing items from an array. Even so, there are two methods you can use, both of which I will explain in this article.
Setting Array Items to Null (Easy Method)
The easiest way to remove an item from a PowerShell array is by setting the item's index position to $Null. The approach makes PowerShell treat that position as if it no longer contains a value. Here's how it works:
First, create an array containing the letters A through F. You can do this by entering this command:
$A=@('A', 'B', 'C', 'D', 'E', 'F')
When you type $A, PowerShell will display the items in the array, as shown in Figure 1.
Figure 1. The array $A contains the letters A through F.
Each element in the array corresponds to a numerical index, starting at 0. For example:
$A[0] is A
$A[1] is B
$A[2] is C
$A[3] is D
$A[4] is E
$A[5] is F
Now, suppose you want to remove the letter D. The simple way to do this is by setting the index associated with D to $Null. Use this command:
$A[3]=$Null
When you type $A again, you will see that the letter D is no longer displayed, as shown in Figure 2
Figure 2. Setting an array position to $Null removes the value from the array.
Given the ease with which I removed an array item, you may wonder why I would even bother explaining the hard way of doing this. The reason why is that we haven’t truly removed the item from the array. Although this method removes the value, it retains the original structure. It continues to have six positions, even though one is empty. For example, typing $A.count will still return 6. Additionally:
A[4] still holds the letter E, and
A[5] still holds the letter F, just as before.
Figure 3. The array position has not been deleted.
In some cases, this method works perfectly fine. However, having empty array positions can cause problems in some situations. If that is the case, you will need a different approach, which is a bit more complicated than what we have so far covered.
Manual Array Reconstruction (Hard Method)
According to Microsoft documentation, adding an item to a PowerShell array involves setting up a brand-new array. Thankfully, PowerShell handles this process automatically. However, removing an item from an array requires you to construct a new array manually. PowerShell doesn’t handle this for you.
Here is how you can fully remove an item from an array:
$A=@('A','B','C','D','E','F')
$A
$Index=0
$TotalItems=$A.count
ForEach ($Item in $A){
If ($Item -eq 'D') {
$Position=$Index
}
$Index=$Index+1
}
Write-Host 'the position is ' $Position
# Copy the letters before D
$B=@($Null)
For ($Counter=0; $Counter -lt $Position; $Counter++) {
$B+=$A[$Counter]
}
#Copy the letters after D
For ($Counter=$Position+1; $Counter -le $TotalItems; $Counter++) {
$B+=$A[$Counter]
}
$A = $B
$A
This script does the same thing we did a moment ago: It extracts the letter D.
The script begins by creating the array in the same way as before. It then sets a variable called $Index to 0 and a variable called $TotalItems equal to the total number of items in the array (6).
A ForEach loop looks at every position in the array to see if its value equals D. When the loop does find the D, it stores its index value in a variable called $Position. In other words, $A[$Position] corresponds to the letter D. While we know in this example that D is in the 3 position, this approach is helpful when the position is unknown.
We create an empty array called $B. I am using two loops to populate the $B array with the data from the $A array. The first loop copies all the data before the letter D (positions 0, 1, and 2). The second loop copies the data after the letter D (positions 4 and 5).
The first of the two loops sets a variable called $Counter equal to 0. It then increments that counter by 1 every time the loop cycles. The loop continues so long as the counter value is less than the $Position variable, which holds the index value for D. Each time the loop cycles, it copies $A[$Counter] to the $B array. In this case, that means that the loop will copy $A[0], $A[1], and $A[2].
The second loop starts with the counter set to $Position + 1, or the position after the letter D. It then copies this position and all other positions (up to the $TotalItems in the array) to the $B array.
At this point, we have created a brand-new array called $B. The array contains all the data from $A except for the letter D. Because it is a new array, D and its position are completely removed.
The last step in the process is to copy the $B array to $A. The r$A array contains all the same data as before, minus the letter D.
Unlike the first method, which sets the target position to $Null but keeps the array structure intact, this method reconstructs the array entirely. As a result:
The letter D and its position are truly gone.
The index values for E and F are updated to reflect the removal of D.
About the Author
You May Also Like