How To Create Functions in Powershell ScriptsHow To Create Functions in Powershell Scripts
PowerShell functions provide a slew of benefits. Here are the basics for designing functions, including functions that use parameters.
June 7, 2022
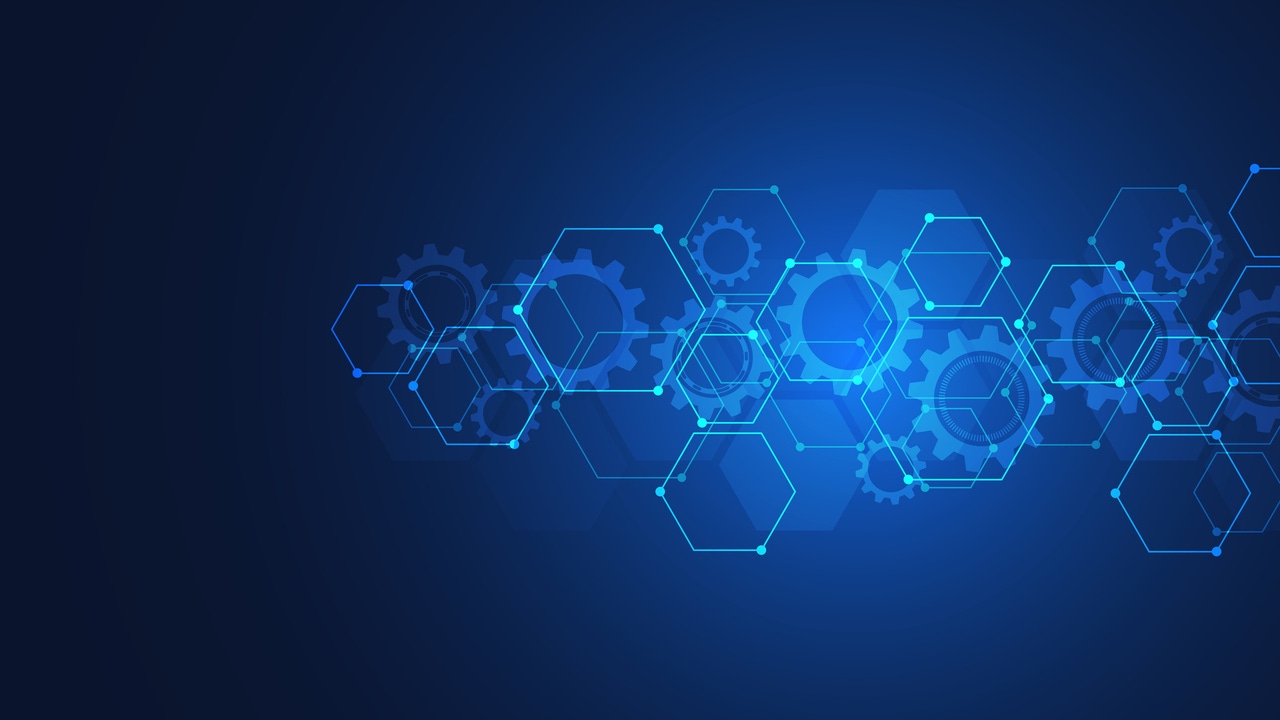
If you write a lot of PowerShell scripts, sooner or later you will find that you need to create a function. PowerShell functions are essentially a block of code that you can run again and again. You can call a PowerShell function as often as you need to.
Benefits of PowerShell Functions
Even if you don’t need to repeatedly run a block of code within a script, PowerShell functions can serve other purposes. Imagine a situation in which you might need to handle an error within a script, but there is no guarantee that an error will occur. A function is perfect in this case because functions provide a way of writing code that will not execute unless called on.
Another benefit of using PowerShell functions is that they can help to make long and complex scripts easier to read and debug. Rather than having the script consist of one huge block of code, you can break major areas of functionality out into functions.
There are countless other benefits to using PowerShell functions. You can even use functions (inside of modules) as a tool for creating custom PowerShell cmdlets!
PowerShell Function Basics
With that said, I want to show you the basics of creating a PowerShell function.
Name your function
The first thing to know about PowerShell functions is that every function must have a name. Technically, you can give a function any name you want, so long as you don’t use any reserved words. However, as a best practice, I recommend giving your functions names that adhere to the basic PowerShell cmdlet structure.
As I’m sure you know, PowerShell cmdlets are usually structured in a verb-noun format. These cmdlets consist of two words, a verb and a noun, separated by a dash. It works well to use the same naming convention for your functions because the verb-noun combination can describe what the function is designed to do.
Additionally, if you later decide to turn the function into a custom PowerShell cmdlet, it will already be in a format that matches the general PowerShell naming convention.
Use ‘Function’
Another thing that you do when you create a PowerShell function is to use the word “Function.” Function appears just before the function name. If you try to declare a function name without using Function, PowerShell will think you have typed an invalid command and give you an error.
Enclose body in brackets
The function body can contain anything that you want. The only real requirement is that the function body must be enclosed in brackets.
Example of a PowerShell Function
Now that I have explained how to declare a function, let’s take a look at a very simple function provided by Microsoft.
The command for finding out which version of PowerShell you are running is $PSVersionTable.PSVersion. This command is not very intuitive, so let’s create a function called Get-Version with the $PSVersionTable.PSVersion command in the function body. That way, you simply type Get-Version to see the PowerShell version.
Here is what the code looks like:
Function Get-Version { $PSVersionTable.PSVersion}
As you can see, we have the word “Function,” followed by a function name and an opening bracket. This is followed by the function body and a closing bracket. Once a function has been declared, you can execute it by entering the function name. You can see what this looks like in Figure 1.
PowerShell Function 1
Figure 1. This is an example of a simple PowerShell function.
PowerShell Functions That Use Parameters
One last thing to show you is how to design a PowerShell function that accepts a parameter (although a PowerShell function can in fact accept multiple parameters).
There are different ways to handle parameters in PowerShell, so let’s look at one of the simpler methods.
The easiest way I know to pass a parameter to a function is to include a variable in parentheses just after the function name. This variable then inherits whatever value is passed to the function. There are far more sophisticated ways of handling parameters, but this method works and is easy to use.
In the following example, I’m going to create a really simple function in which I pass a text string to the function, then use the function to display the contents of that texturing.
Here is what the function looks like:
Function Display-Text($MyText){ Write-Host $MyText}
This function, which you can see in Figure 2, accepts a string of text as input and then uses the Write-Host cmdlet to display that text. I supply the text immediately after entering the function name when I call the function.
PowerShell Function 2
Figure 2. I have passed a value to a function.
You will notice that I have called the function twice. The first time, I just passed a raw text string to the function. The second time, I added the text string to a variable and passed the variable to the function. Both techniques produced the same output.
About the Author
You May Also Like