An Explorer's Guide to Discovering and Using PowerShell MethodsAn Explorer's Guide to Discovering and Using PowerShell Methods
Finding out how to use methods on a PowerShell object, even if the docs don't exist!
June 23, 2015
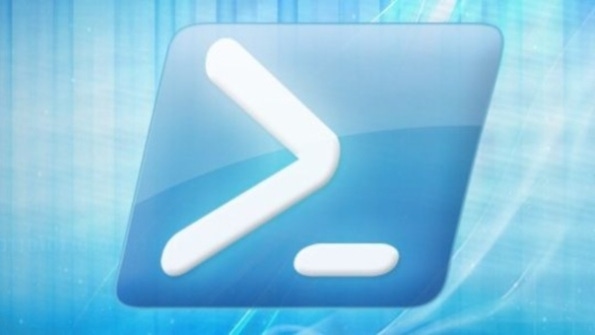
Most truly powerful Windows power tools are command-line and scripting tools. However, many administrators still aren’t using them. The primary reasons? They’re hard to find, and they’re hard to figure out. Anyone who read last month’s column, “Exploring Useful .NET Classes with PowerShell to Add Speech” (which was about using the New-Object command to create an object that could make your PC speak text) might have come away saying, “OK, that was nice, but how did you discover all that, and how could I have figured it out by myself?” This month, I’ll show you two easy methods for figuring out how .NET objects and their methods work, so that you can use them in your PowerShell activities.
A Quick Review
You’ve previously seen methods and their syntax—for example, in “Using PowerShell Object Methods.” Remember how the AddDays method on Get-Date lets you see the date 2000 days from now?
(get-date).AddDays(2000)
PowerShell objects have methods, as well as actions you can perform on them, and their syntax looks like object.methodname(arguments-to-pass-to-the-method). Further, many methods don’t need arguments, but they need the parentheses anyway, like (get-date).IsDaylightSavingTime(). You’ve also seen that you can discover any object’s methods with Get-Member, as in (get-date) | get-member -MemberType Method.
Last month, I took things a bit further and created an object that lets PowerShell speak via a .NET class called SpeechSynthesizer:
Add-Type -AssemblyName System.speech$talker = New-Object System.Speech.Synthesis.SpeechSynthesizer$talker.speak(“hello there”)
I suggested typing
$talker | get-method -MemberType Method
to show the methods, but the output was a bit minimal. Several methods—Speak, SpeakAsync, SetOutputToWaveFile, SetOutputToDefaultAudioDevice, SelectVoice, SelectVoiceByHints, and GetInstalledVoices—looked potentially useful. How might you find out more about, for example, SetOutputToWaveFile?
List the Overloads
Your first approach should be to “dump the overloads.” So, just type the method name without parentheses, like this:
PS C:> $talker.SetOutputToWaveFileOverloadDefinitions-------------------void SetOutputToWaveFile(string path)void SetOutputToWaveFile(string path, System.Speech.AudioFormat.SpeechAudioFormatInfo formatInfo)
That’s valuable because it tells you what kinds of data this method requires, as well as whatever name the programmer gave that desired input—helpful clues! String is the type of data required, and path is the name that the programmer assigned to it. Armed with that information, you can guess that this requires a full file specification (path and name) to function properly. Notice also that there are two ways to call this method: with only the path value, or with the path value and some kind of data that specifies how to encode the sound (e.g., sampling rates, compression algorithm). How about trying it with just a file specification?
$talker.SetOutputToWaveFile("C:scriptstest2.wav")
If you then do a few more $talker.speak(whatever) commands, Windows creates the Test2.wav file and fills it with that speech. Hmmm. Nothing from the speakers yet.
You can create WAV files containing whatever text you want! So, to redirect the speech output back to the speaker, it looks like you can use the SetOutputToDefaultAudioDevice method. Again, what sort of arguments does this method need? Type $talker.SetOutputToDefaultAudioDevice, press Enter, and you’ll get just one OverloadDefinition:
void SetOutputToDefaultAudioDevice()
There’s nothing between the parentheses, so all you need to do to make it work is to just type
$talker.SetOutputToDefaultAudioDevice()
And from that point on, $talker only talks out of the speaker.
Consult the Developer Docs
Recall that last month’s column explored GetInstalledVoices. If you just type $talker.GetInstalledVoices without the parentheses, it returns two overloads: a simple pair of parentheses and (cultureinfo). What is cultureinfo, and how might it be useful? To find out, you can use another method: Read the developer docs.
You know that the .NET class name is SpeechSynthesizer, so you can Google “Windows SpeechSynthesizer class,” which leads you to an MSDN page entitled “SpeechSynthesizer Class (System.Speech.Synthesis) – MSDN.” That page contains a link called SpeechSynthesizer Methods. Clicking that link gets you a table of methods, including GetInstalledVoices Method (CultureInfo).
That page starts off with some remarks that offer examples of cultureinfo, like en-US. Aha! You’ve seen those abbreviations before. In my case, I know my Windows 8.1 system shipped with two American voices and a British voice, so I try out
$talker.GetInstalledVoices("en-gb").voiceinfo.name
(Consult last month’s article for a reminder about why I ended it with .voiceinfo.name.) The result is that it returns just one voice: Microsoft Hazel Desktop.
You’ve seen the two primary approaches to prying loose information about using a method: List its overloads and consult the MSDN docs. But I can’t finish this article without briefly covering the first option that everyone reaches for: a simple Google search. Yes, Googling “PowerShell .NET speechsynthesizer method examples” will yield some very nice information uncovered by others, but you’ll often miss out on the things that they didn’t find or didn’t think interesting enough to report. Happy exploring!
About the Author
You May Also Like