Advanced String Manipulation Techniques in PowerShell
Explore these string manipulation techniques, including converting strings to arrays, inserting and removing text, and correctly using single and double quotes, to dynamically construct commands.
June 28, 2024
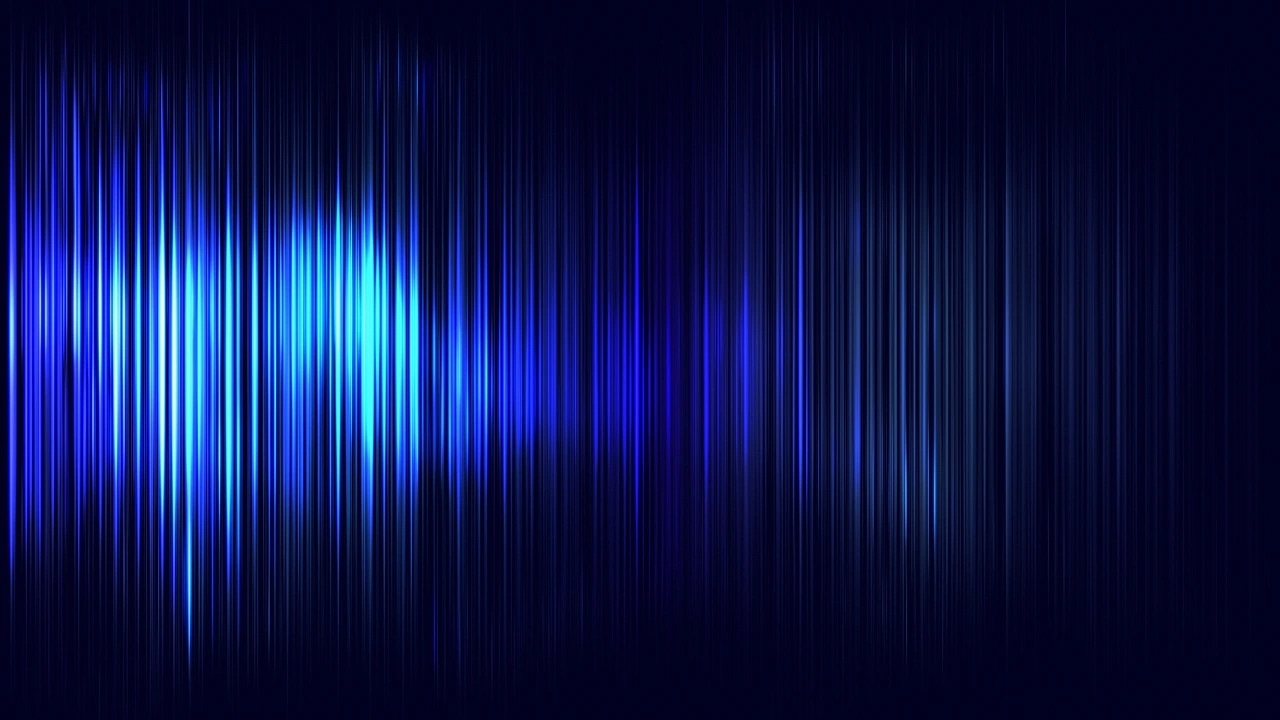
In a previous article, I explained the need for string manipulation when dynamically generating PowerShell commands. We examined declaring and concatenating strings. Here, I wanted to share a few more techniques that I commonly use:
Turning a String Into an Array
Inserting and Removing Portions of a String
Single vs. Double Quotes Revisited
Turning a PowerShell String Into an Array
One technique for building commands dynamically is converting a string into an array. The conversion to an array makes it easy to extract and manipulate portions of the string individually.
For example, in my previous article, I created a variable called $String3 containing the words “This is an example of joining two strings together.” To isolate the word example, you can do the following:
$String4 = $String3 -Split “ “
$String4[3]
In this example, I have set $String4 equal to $String3 with a split. I specified a space as the delimiter, meaning the string will split into individual words. Hence, $String4 becomes an array containing all the individual words from $String3. I isolated the word example by referencing its position within the array. You can see what this looks like in Figure 1.
Figure 1. I have converted a text string into an array to pick out individual words.
Inserting and Removing Portions of a String
Another technique is to modify a string by removing or inserting content. Both operations are easy to do.
Removing characters from a string
As a demonstration, we’ll create a string called $String and set its value to the words “This is a string manipulation technique”. Here is the command:
$String= “This is a string manipulation technique”
If we wanted to remove the word “manipulation,” we first count the characters up where “manipulation” starts (the 17th character) and then remove 13 characters (12 for the word “manipulation” and 1 for the space after the word).
$String = “This is a string manipulation technique”
$NewString = $String.Remove(17,13)
You can see the results in Figure 2.
Figure 2. I have removed the word “manipulation” from $String.
Inserting characters into the string
To insert the word “manipulation” (with a space following the word) back into $String at the 17th position, you can use the following code:
$String=“This is a string technique”
$NewString=$String.Insert(17,”manipulation “)
You can see the results in Figure 3.
Figure 3. I have added the word “manipulation” back into $String.
Single vs. Double Quotes Revisited
In my previous article on string manipulation, I briefly touched on the issue of single (‘ ’) and double (“ ”) quotation marks. Use quotation marks correctly to avoid errors when dynamically constructing PowerShell commands.
For example, it would matter which type of quotation marks you used when dynamically constructing a command that displays the contents of our $String variable using the Write-Host cmdlet. While we could display the variable’s contents without using Write-Host, I want to demonstrate a common issue with this cmdlet.
Usually, when using the Write-Host cmdlet, you enclose the text to be displayed within quotation marks. However, if you include quotation marks within a string, PowerShell interprets them as the end of the string. The solution is to enclose single quotation marks inside of double quotation marks and vice versa. Here is an example:
$String=“This is a string technique”
$NewString = “Write-Host “ ++ “’” + $String + “’”
Notice how this example uses the string concatenation method discussed in the previous article. The figure below illustrates the results of these commands.
Figure 4. I have dynamically constructed and executed a PowerShell command.
Learn more about PowerShell strings:
About the Author
You May Also Like