Rendering Data on the Client with jQuery TemplatesRendering Data on the Client with jQuery Templates
Reduce coding time by putting the powerful jQuery Templates plug-in to work in your ASP.NET applications
April 13, 2011
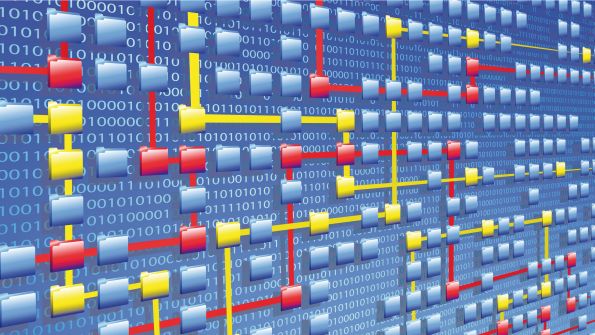
To minimize the amount of code that has to be written for an application, nearly every programming language uses templates in some manner. You can define a template structure one time and use it to generate code, HTML, or other formats. If you've created ASP.NET applications, you already know how powerful and productive templates are when it comes to rendering HTML. For example, you may have used an item template similar to the following in a ListView or other type of server control:
<%#Eval("CustomerID") %> <%#Eval("CompanyName") %>
Wouldn't it be nice if you could follow the same process on the client side? Well, if you're working with JavaScript Object Notation (JSON) objects, you certainly can.Although templates have been available in script libraries for some time through the use of various plug-ins, the latest template framework that was created jointly by Microsoft and the jQuery team provides a great solution that doesn't use Cascading Style Sheets (CSS) in strange ways or require a lot of knowledge about a template language. By using this framework, you can define HTML templates in web pages and use them to render HTML output dynamically at runtime simply by passing a JSON object into a template function. This process is extremely easy to use and very productive. Ultimately, it also means there's much less JavaScript code that you have to write and maintain.
Defining a Template Block
To get started using jQuery template functionality, you'll need to download the jquery.tmpl.js script plug-in files, available on the GitHub Social Coding website. (The plug-in was in beta when this article was being written.) To define a template, you reference the template script in your web page, then define a
After you define the script tag, you can put template code within it. Any HTML that you add is output automatically after the template is rendered. Of course, adding static HTML doesn't accomplish much. Therefore, the jQuery Templates plug-in provides a template tag language. This language contains several tags that you can add to a template to perform many different tasks, such as defining data that should be generated as output, performing conditional logic, iterating through items, and rendering nested templates.
jQuery Templates TagsThe jQuery Templates plug-in defines several different tags that can be embedded in a template and that are used to control the type of data rendered. I'll introduce you to the different template tags that are available, and demonstrate how to use them.
${ PropertyName } tag. This is arguably the most useful tag you'll use within a template, and one that you'll find yourself using over and over. This tag is similar to the <%# Eval("PropertyName") %> data-binding expression that you use to bind properties within ASP.NET server control templates. This tag is responsible for evaluating the specified property on the current data item and for writing out the property. You can also wrap a JavaScript function around the property in situations in which the data must be manipulated in some way. The following code shows this tag in use:
If the value of the DeliveryFee property is $5.00, for example, the output is generated as follows after the template is rendered:
$5.00
{{ html PropertyName }} tag. This tag is used to handle data that may contain HTML markup, such as My Comment. Whereas the ${ PropertyName } template tag encodes data, the {{ html PropertyName }} tag doesn't encode the text as it's added into the HTML Document Object Model (DOM). The following code shows this tag in use:
{{ if }} tag. This tag is fairly common, and it's especially useful if you have to generate output data or markup that's based on one or more conditions. This tag can be used to evaluate a condition and render content, as appropriate. As with most programming languages, the {{ if }} tag can be associated with an {{ else }} tag in cases where multiple conditions are tested. Figure 1 shows this tag in use.
{{if MainItems.length==0}} No items selected {{else}} Ordered items! {{/if}}
{{ each }} tag. This tag is used to perform looping operations within a template. For example, you may have a JSON object that contains order information, and the order information contains an array of ordered items named MainItems. You can iterate through each item by using the {{ each }} tag. The code in Figure 2 shows that the {{ each }} tag accepts an index position (i), a variable name for the current item (mi), and the name of the array to iterate through. The variable name (mi) can be used to access properties of the current item. This example renders the NumberOrdered and Price property values within a table cell.
{{each(i,mi) MainItems}} ${ mi.NumberOrdered } ordered at $ ${ mi.Price} per item {{/each}}
{{tmpl}} tag. This tag is used to reference one template from within another, thereby allowing template reuse. In the example shown in Figure 3, OrderSummaryTemplate references MainItemsTemplate by using the {{tmpl '#MainItemsTemplate'}} syntax.
The result is that one or more rows from MainItemsTemplate are embedded directly below the Items Ordered row within OrderSummaryTemplate. By using this technique, you can more easily reuse templates within an application or break up large templates into more manageable pieces.
{{wrap}} tag. This tag is similar to the {{ tmpl }} tag in that it lets you embed one template within another. But it also provides a way to wrap content around a specific template's content. The jQuery documentation provides an elegant yet simple example of using {{ wrap }}, which is shown in Figure 4.
At first glance, it may appear that the {{ wrap }} tag and the {{ tmpl }} tag are identical because both use similar syntax. However, you can see in the code in Figure 4 that the template contains a closing {{/wrap}} tag. In this example, the two
tags inside the myTmpl template will be wrapped inside the tableWrapper template. This means that each tag will be placed inside a tag. The tableWrapper template handles the placement of each tag inside a tag by using the $item.html('div') call within an {{ each }} loop. This puts each tag inside the {{ wrap }} and {{ /wrap }} tags, and it also puts the tag inside a tag by using {{html $value}}.
Rendering a Template
After a template is defined by using a
About the Author
You May Also Like