Create load balancer with NAT rules pointing to VMs using PowerShell
Use a load balancer with ARM and AzureRM PowerShell to point to VMs
January 13, 2016
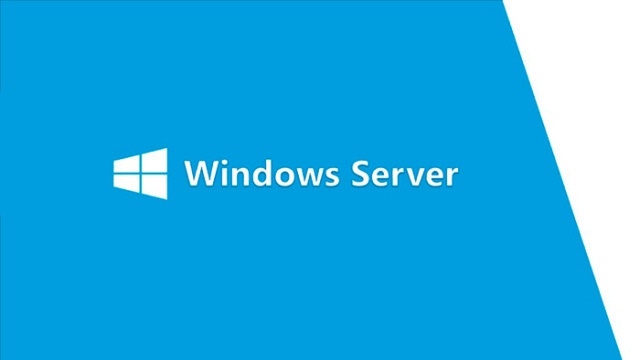
Q. How do I create a load balancer with NAT rules pointing to VMs using PowerShell with AzureRM?
A. With Azure Resource Manager (ARM), IaaS cloud services are not used which with Azure Service Manager (ASM) provided a Virtual IP that via endpoints could provide connectivity to VMs from the Internet. With the absence of the cloud service and its VIP there are two approaches to connecting to VMs from the Internet:
Give the VM a Public IP address making it directly communicable from the Internet
Create an external load balanced (a load balancer with a public IP address) that uses NAT rules to directly pass traffic to ports on VMs or load balancing rules to distribute traffic between VMs
Remember both of these approaches should only be used when you need connectivity from the Internet. Most normal communications would occur from on-premises to Azure connections such as site-to-site VPN and ExpressRoute.
The first option is very simple to do, simply create a Public IP (PIP) and assign to the network adapter that will be used with the VM. For example:
$pip = New-AzureRmPublicIpAddress -ResourceGroupName $rgname -Name "pip1" `-Location $loc -AllocationMethod Dynamic -DomainNameLabel $vmname.ToLower()$nic = New-AzureRmNetworkInterface -Force -Name ('nic' + $vmname) -ResourceGroupName $rgname `-Location $loc -SubnetId $subnetId -PublicIpAddressId $pip.Id
To use a load balancer there are numerous options and Microsoft has a great walkthrough at https://azure.microsoft.com/en-us/documentation/articles/load-balancer-internal-arm-powershell/#create-lb-rules-nat-rules-probe-and-load-balancer and https://azure.microsoft.com/en-us/documentation/articles/virtual-machines-ps-create-preconfigure-windows-resource-manager-vms/. Below is some simple PowerShell that creates a load balancer only for use with NAT rules to forward traffic and therefore does not use a health probe or normal load balancer objects.
$pipLB = New-AzureRmPublicIpAddress -ResourceGroupName $rgname -Name "vipLB" `-Location $loc -AllocationMethod Dynamic -DomainNameLabel "viplb"$frontendIP = New-AzureRmLoadBalancerFrontendIpConfig -Name LB-Frontend -PublicIpAddressId $pipLB.Id$inboundNATRule1= New-AzureRmLoadBalancerInboundNatRuleConfig -Name "RDP1" ` -FrontendIpConfiguration $frontendIP -Protocol TCP -FrontendPort 3441 -BackendPort 3389$NRPLB = New-AzureRmLoadBalancer -ResourceGroupName $rgname -Name "NRP-LB" ` -Location $loc -FrontendIpConfiguration $frontendIP -InboundNatRule $inboundNATRule1
Notice that this load balancer uses a public IP address for its front end IP then has a single NAT Rule that will forward traffic from port 3441 to a backend port of 3389 (RDP). You can add multiple NAT rules just by creating multiple NAT rule objects and including them in the load balancer, e.g. -InboundNatRule $inboundNATRule1,$inboundNatRule2,etc.
To use the NAT rule created when creating the NIC use:
$nic = New-AzureRmNetworkInterface -Force -Name ('nic' + $vmname) -ResourceGroupName $rgname ` -Location $loc -SubnetId $subnetId -LoadBalancerInboundNatRule $NRPLB.InboundNatRules[0]
Looking at the properties of the NIC will show the NAT rule. For example:
Name : nictestvmARM2ResourceGroupName : RG-SCUSALocation : southcentralusId : /subscriptions/466c1a5d-e234-1234-91a5-x234234x0f8/resourceGroups/RG-SCUSA/providers/Microsoft.Networ k/networkInterfaces/nictestvmARM2Etag : W/"4f3e04bb-16b6-4330-83de-a0879b9a4784"ResourceGuid : f974a6d1-00b3-40ba-952d-b12026e9baceProvisioningState : SucceededTags : VirtualMachine : nullIpConfigurations : [ { "Name": "ipconfig1", "Etag": "W/"4f3e04bb-16b6-4330-83de-a0879b9a4784"", "Id": "/subscriptions/466c1a5d-e234-1234-91a5-x234234x0f8/resourceGroups/RG-SCUSA/providers/Micro soft.Network/networkInterfaces/nictestvmARM2/ipConfigurations/ipconfig1", "PrivateIpAddress": "10.0.0.5", "PrivateIpAllocationMethod": "Dynamic", "Subnet": { "Id": "/subscriptions/466c1a5d-e234-1234-91a5-x234234x0f8/resourceGroups/RG-SCUSA/providers/Mic rosoft.Network/virtualNetworks/vnetRG-SCUSA/subnets/subnetRG-SCUSA", "IpConfigurations": [] }, "PublicIpAddress": { "Id": "/subscriptions/466c1a5d-e93b-4138-91a5-670daf44b0f8/resourceGroups/RG-SCUSA/providers/Mic rosoft.Network/publicIPAddresses/vip1" }, "ProvisioningState": "Succeeded", "LoadBalancerBackendAddressPools": [], "LoadBalancerInboundNatRules": [ { "Name": "RDP1", "Etag": "W/"a12a241c-cec4-4111-bd9b-a7db113f03e9"", "Id": "/subscriptions/466c1a5d-e234-1234-91a5-x234234x0f8/resourceGroups/RG-SCUSA/providers/M icrosoft.Network/loadBalancers/NRP-LB/inboundNatRules/RDP1", "FrontendIPConfiguration": { "Id": "/subscriptions/466c1a5d-e234-1234-91a5-x234234x0f8/resourceGroups/RG-SCUSA/providers /Microsoft.Network/loadBalancers/NRP-LB/frontendIPConfigurations/LB-Frontend" }, "Protocol": "Tcp", "FrontendPort": 3441, "BackendPort": 3389, "IdleTimeoutInMinutes": 4, "EnableFloatingIP": false, "ProvisioningState": "Succeeded" } ] } ]DnsSettings : { "DnsServers": [], "AppliedDnsServers": [] }EnableIPForwarding : FalseNetworkSecurityGroup : nullPrimary : False
Note in the example above the NIC has both a public IP and a NAT rule meaning the VM can be accessed from the Internet 2 different ways.
Note you can also use JSON templates which is the preferred approach. Microsoft has examples using templates at https://github.com/Azure/azure-quickstart-templates with a write-up at https://azure.microsoft.com/en-us/documentation/templates/101-internal-loadbalancer-create/. https://github.com/Azure/azure-quickstart-templates/blob/master/101-vm-with-rdp-port/azuredeploy.json includes the VM creation, load balancer creation with a PIP and the NAT rule.
About the Author
You May Also Like