While most tasks involving arrays in PowerShell are relatively easy, removing an item from an array presents a challenge. PowerShell lacks a native cmdlet designed specifically for removing items from an array. Even so, there are two methods you can use, both of which I will explain in this article.
Method #1: The Easy Way
The easy way of removing an item from a PowerShell array is to set the item's index position to $Null. This action essentially makes PowerShell treat the position as if it no longer exists. Let me show you how this works.
To get started, let’s create an array containing the letters A through F. You can do this by entering this command:
$A=@(‘A’, ‘B’, ‘C’, ‘D’, ‘E’, ‘F’)
Typing $A causes PowerShell to display the items in the array, as shown in Figure 1.

Figure 1. The array $A contains the letters A through F.
It’s important to understand that each element in an array (represented by the letters A through F) corresponds to a numerical index value. The indexing starts at 0, meaning that $A[0] is equal to A, $A[1] is equal to B, and so forth. Since there are six letters stored in the array, the index values range from 0 to 5, where $A[5] contains the letter F.
With that in mind, let’s suppose that we want to remove the letter D from the array. The simple way to do this is to set the index position associated with the letter D to $Null. Here’s the command:
$A[3]=$Null
If you type $A to display the array’s contents now, the letter D is gone, as shown in Figure 2.

Figure 2. Setting an array position to $Null removes the value from the array.
Given the ease with which I just removed an array item, you may be wondering why I am even going to bother explaining the hard way of doing this. The reason why is that we haven’t truly removed the item from the array. Although the value is gone, the array still retains six positions (you can check by typing $A.count). Additionally, the letter E remains in the 4th index position, and F is still in the 5th index position, just as it was before I removed the letter D.

Figure 3. The array position has not been deleted.
In some cases, the method that I just showed you may be perfectly fine. In other cases, having empty array positions can really cause problems. This is why I will explain the hard way of removing an item from an array.
Method #2: The Hard Way
According to Microsoft documentation, adding an item to an array involves creating a brand-new array. Thankfully, PowerShell handles this process seamlessly. However, when it comes to truly removing an item from an array, you also must construct a brand-new array. The difference is that PowerShell doesn’t automatically handle this.
Here is how you can truly remove an item from an array:
$A=@('A','B','C','D','E','F') $A $Index=0 $TotalItems=$A.count ForEach ($Item in $A){ If ($Item -eq 'D') {$Position=$Index} $Index=$Index+1 } Write-Host 'the position is ' $Position # Copy the letters before D $B=@($Null) For ($Counter=0; $Counter -lt $Position; $Counter++) { $B+=$A[$Counter] } #Copy the letters after D For ($Counter=$Position+1; $Counter -le $TotalItems; $Counter++) { $B+=$A[$Counter] } $A = $B $A
This script does the same thing that we did a moment ago: It removes the letter D from the array.
The script starts by creating the array in the same way as before. It then sets a variable called $Index to 0 and sets a variable called $TotalItems equal to the total number of items in the array (6).
Next, a ForEach loop looks at every position in the array to see if its value is equal to D.
When the loop does find the letter D in the array, it stores its index value in a variable called $Position. In other words, $A[$Position] corresponds to the letter D. Being that we already know that the letter D is in the 3 position, we could have just set $Position to be equal to 3 and not bothered creating this loop. In real-world scenarios, however, the position is often unknown.
Next, I have created an empty array called $B. I am using two loops to populate the $B array with the data from the $A array. The first loop copies all the data that comes before the letter D (positions 0, 1, and 2). The second loop copies the data that comes after the letter D (positions 4 and 5).
The first of the two loops sets a variable called $Counter equal to 0. It then increments that counter by 1 every time the loop cycles. The loop continues so long as the counter value is less than the $Position variable, which holds the index value for D. Each time the loop cycles, it copies $A[$Counter] to the $B array. In this case, that means that the loop will copy $A[0], $A[1], and $A[2].
The second loop starts with the counter set to $Position + 1, or the position after the letter D. It then copies this position and all other positions (up to the $TotalItems in the array) to the $B array.
At this point, we have created a brand-new array called $B. The array contains all the data from $A except for the letter D. Because it is a new array, D and its position are truly gone.
The last step in the process is to copy the $B array to $A. The result is that the $A array contains all of the same data as before, minus the letter D. Unlike the first method I explained, the value D is truly removed from the array along with its position, and the index values for E and F have changed to reflect the removal of D.
About the author
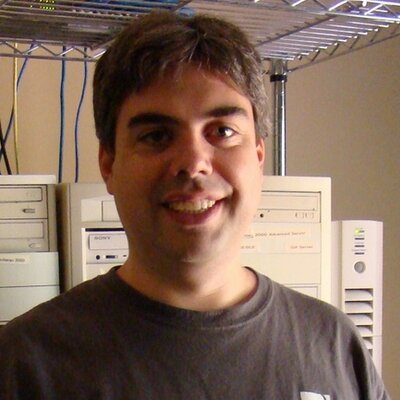