When using PowerShell, it can be helpful to write a script’s output to a file instead of just viewing it on the screen.
For instance, I’ve created complex PowerShell scripts in the past that generate logging data when certain events occur. While running the script, I don’t necessarily care to see this logging data on the screen, but having a log file can be valuable for reference in the case of unexpected events.
As another example, I’ve built PowerShell scripts that gather system information and save it to a file. One of these is a disk health tool that generates a file containing health information for all the disks I use.
The bottom line is that there are millions of situations where you might need a PowerShell script to create a text file. However, the techniques required for creating a script can vary widely based on the specific goal you’re trying to achieve.
Basic Method for Creating and Appending a Text File
Simple file creation
Creating a basic text file is really simple. You can use the Out-File cmdlet followed by the path and name of the file you want to create.
Let’s say you want to generate a text string with the words “Hello from PowerShell” and write it to a file named Example.txt. You can achieve this with the following commands:
$String=“Hello from PowerShell” $String | Out-File -FilePath ‘C:\Temp\Example.txt’

Figure 1. I created a file named example.txt and used the Get-Content cmdlet to display the file’s contents.
Appending to existing files
If you want to add the phrase “Hello World” to the existing file, simply repeating the previous commands with “Hello World” instead of “Hello from PowerShell” would not work. If you did that, the new text would overwrite the old text. This is demonstrated in Figure 2.

Figure 2. The file has been overwritten.
To append the new text to the file, you can use the Add-Content command instead of the Out-File command. Here’s an example of how you can create a file and then append text to it:
$String=“Hello from PowerShell” $String | Out-File -FilePath ‘C:\Temp\Example.txt’ $String=“Hello World” $String | Add-Content ‘C:\Temp\Example.txt’

Figure 3. I have added a line of text to a file.
Real-World Applications
Okay, so that was a nice demo, but why would you ever need to use this technique in the real world? Well, consider a script that generates log files where the filename is based on the current date. The script starts by creating an empty file and then appends data each time logging information is generated.
In such a script, you’re not just writing static text to a file as in the previous example. Instead, the script uses string manipulation to create uniformly formatted logging data. This highlights an important point: When formatting output data in PowerShell, you aren’t restricted to string concatenation alone; you can also take advantage of escape codes for formatting.
Using Escape Codes for Formatting
Escape codes in PowerShell apply special formatting to a string. To include an escape code, use the backward apostrophe (or backtick, found just to the left of the 1 key on a standard keyboard) followed by the number or letter associated with the code you want to use. It’s important to note that escape codes work only in strings designated with double quotation marks – e.g., $String=“Hello World”. They do not apply to strings using single quotation marks, such as $String=‘Hello World’.
Microsoft provides a list of escape codes here. Some of the more useful escape codes include the following:
`0 Null `a Alert (Causes the system to make a noise) `b Backspace `n New Line `t Tab
Example: tabbed data
Let’s suppose that we are creating a PowerShell log file where you want to display three distinct pieces of data on each row. You can do this with the following code:
$String=”Data1`tData2`tData3” $String | Out-File -FilePath ‘C:\Temp\Example.txt’ $String=”Data4`tData5`tData6” $String | Add-Content ‘C:\Temp\Example.txt’ $String=”Data7`tData8`tData9” $String | Add-Content ‘C:\Temp\Example.txt’ Get-Content Example.txt
In this case, we are simply using words like “Data1” and “Data4” as placeholders for actual data. Even so, this example clearly illustrates that using the `t escape code prompts PowerShell to insert a tab before a piece of data. You can see the result in Figure 4.

Figure 4. The use of an escape code has produced tabbed data.
Of course, we could achieve the same outcome by creating an object and then exporting the object to a text file. However, using escape characters is a handy alternative in situations where the output can widely vary, making the use of an object impractical.
About the author
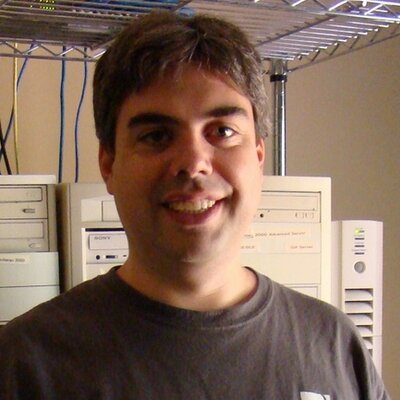