PowerShell scripts typically run until they finish, but it can be useful to build in a mechanism for the script to terminate gracefully after completing its intended tasks.
Exiting a Text-Based Script
If your script is text-based, adding a termination mechanism is super simple. Just using the command Exit in PowerShell will prompt the script to terminate.
For example, suppose that you had a script containing these commands:
Write-Host ‘Line 1’ Write-Host ‘Line 2’ Exit Write-Host ‘Line 3’
The script would display the phrases “Line 1” and “Line 2”, but it would never display “Line 3” because the exit command causes the script to terminate before it ever reaches the “Line 3” command.

Figure 1. The last line of code does not execute because the script terminates before reaching that point.
Of course, you wouldn’t typically insert an Exit command at a random point in a PowerShell script. Instead, you might use the Exit command based on user input. For example, you could present a prompt like, “Press Y to continue or N to exit”.
Here is what the code used to create such a prompt might look like:
$Prompt = Read-Host 'Do you want to continue? Press Y for Yes or N to exit' Switch ($Prompt) { Y {Write-Host 'The script is continuing'} N {Exit} } Write-Host 'The script executes yet another instruction'
In this case, the Read-Host command prompts the user for input. If the user presses Y, then a couple of Write-Host commands will execute. If the user presses N, the script terminates.

Figure 2. You can create a prompt that gives the user the option of exiting the script.
Exiting a GUI-Based Script
As you can see, terminating a text-based script is simple – just insert the Exit command at the desired termination point. However, let’s look at how you can terminate a GUI-based script.
Here is the source code for a simple GUI-based script:
Add-Type -AssemblyName System.Windows.Forms # Create the form $form = New-Object Windows.Forms.Form $form.Text = “Hello World Popup” $form.Size = New-Object Drawing.Size(500, 500) $form.FormBorderStyle = [Windows.Forms.FormBorderStyle]::FixedDialog $form.StartPosition = [Windows.Forms.FormStartPosition]::CenterScreen $Form.KeyPreview = $True $Form.Add_KeyDown({if ($_.KeyCode -eq “Escape”) {$Form.Close()}}) # Create the label to display “Hello World” $label = New-Object Windows.Forms.Label $label.Text = “Hello World” $label.Font = New-Object Drawing.Font(“Arial”, 14, [Drawing.FontStyle]::Bold) $label.AutoSize = $true $label.Location = New-Object Drawing.Point(100, 40) $label.ForeColor = [System.Drawing.Color]::Black $Button1 = New-Object System.Windows.Forms.Button $Button1.Location = New-Object System.Drawing.Size (200,250) $Button1.Size = New-Object System.Drawing.Size(80,30) $Button1.Font=New-Object System.Drawing.Font(“Lucida Console”,14,[System.Drawing.FontStyle]::Regular) $Button1.BackColor = “LightGray” $Button1.Text = “Exit” $Button1.Add_Click({ $Form.Close() }) # Add the label to the form $form.Controls.Add($label) $Form.Controls.Add($Button1) # Display the form $form.ShowDialog()
This script displays the words Hello World within a GUI interface. The only thing that makes it different from any other Hello World script that you might have seen before is that the GUI also contains an exit button, as shown in Figure 3.

Figure 3. This simple Hello World script contains an exit button.
At a functional level, this script is straightforward and works just like any other PowerShell GUI-based script. I began by loading the necessary assemblies. From there, I created a form object, a label object (the Hello World text), and a button object. Then, I told PowerShell to add the label object and the button object to the form and to display the form.
So, now that I have outlined how the script works, let’s examine the exit button. Whenever you create a button object in PowerShell, you have the option of associating a click action with the button. Here are the commands associated with the click action:
$Button1.Add_Click({ $Form.Close() })
In other words, when a user clicks the Exit button, PowerShell simply executes an instruction to close the form. Normally, this is sufficient to terminate the script, but if you have created a highly complex script that runs a lot of non-GUI-related instructions, you may also have to include the exit command just before the closing brackets. Doing so would look something like this:
$Button1.Add_Click({ $Form.Close() Exit })
Escape Key Termination
There’s an additional feature in the script that I haven’t discussed yet. I have configured it to terminate if the user presses the escape key on the keyboard. This functionality is implemented through three lines of code in the section where the form object is created. The following are the three lines of code:
$Form.KeyPreview = $True $Form.Add_KeyDown({if ($_.KeyCode -eq "Escape") {$Form.Close()}})
The first line tells the form to watch for key presses. You will notice that this is a function of the form itself, not an object on the form. In other words, there’s no need to prompt user input or create an input-related object.
The second line tells PowerShell which key we are interested in. In this case, we want to take action if the user presses the Escape key.
The third line tells PowerShell what to do if the escape key is pressed. Once again, we are going to close the form.
As you can see, there are any number of different ways that you can gracefully terminate a PowerShell script. The technique that you use largely depends on whether the script is text-based or GUI-based.
About the author
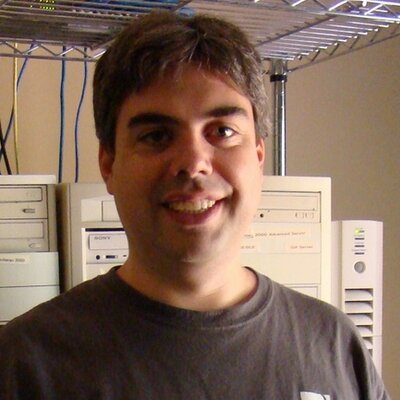